アプリ管理
アプリ管理機能はアプリケーション全体の設定を管理する基本的な仕組みを提供します。
このページでは、アプリ管理機能を利⽤する実装例を紹介します。
アプリケーション設定を編集する
管理画面の「アプリ管理」→「アプリケーション設定」を開くと、アプリケーション設定を編集することができます。
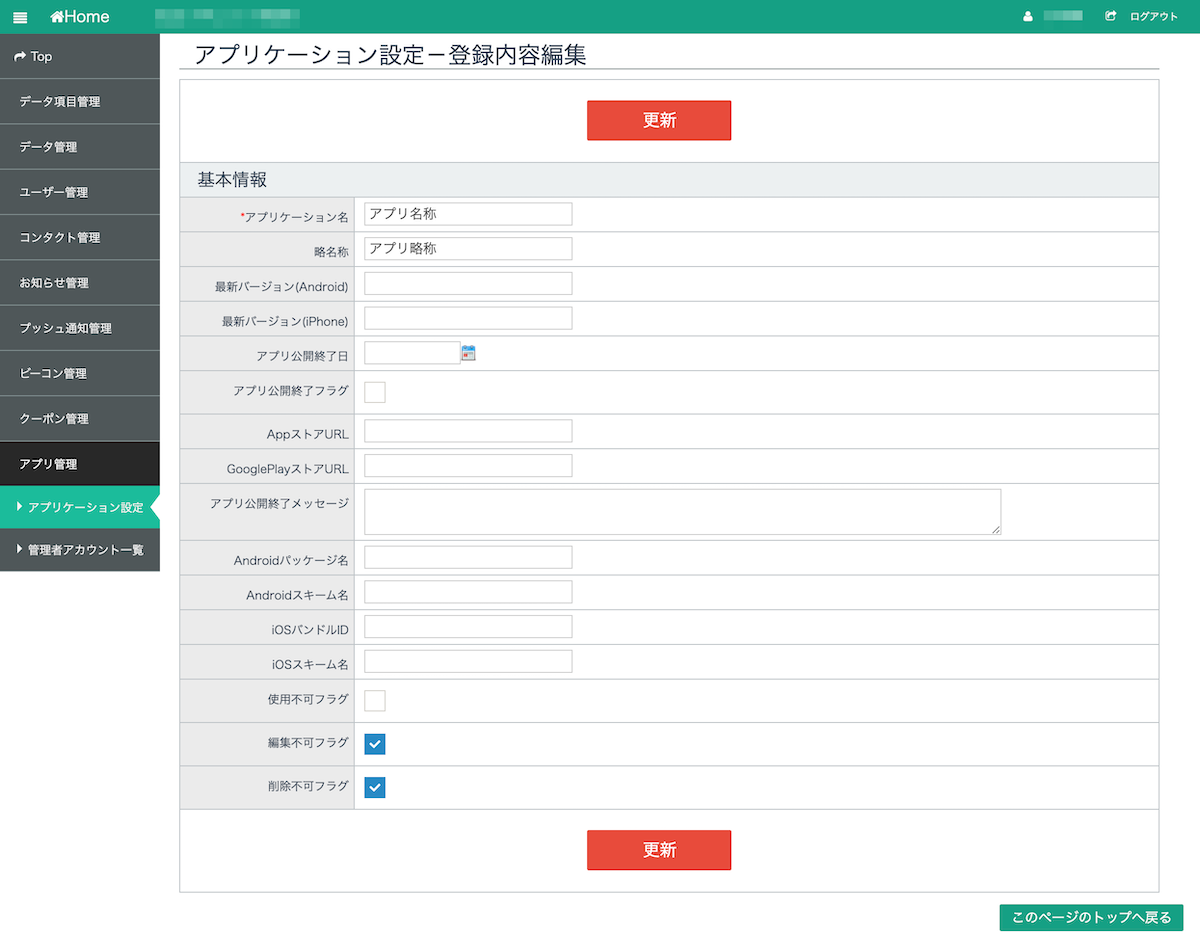
標準でいくつかの項目がありますが、アプリケーション独自の項目を設定することもできます。
アプリケーション独自の項目を設定するには、管理画面の「データ項目管理」→「各種オブジェクト項目設定」を開き、一覧から「アプリケーション設定」を探して「項目設定」をクリックしてください。
項目の設定方法はデータ管理機能のオブジェクト項目を設定すると同様です。
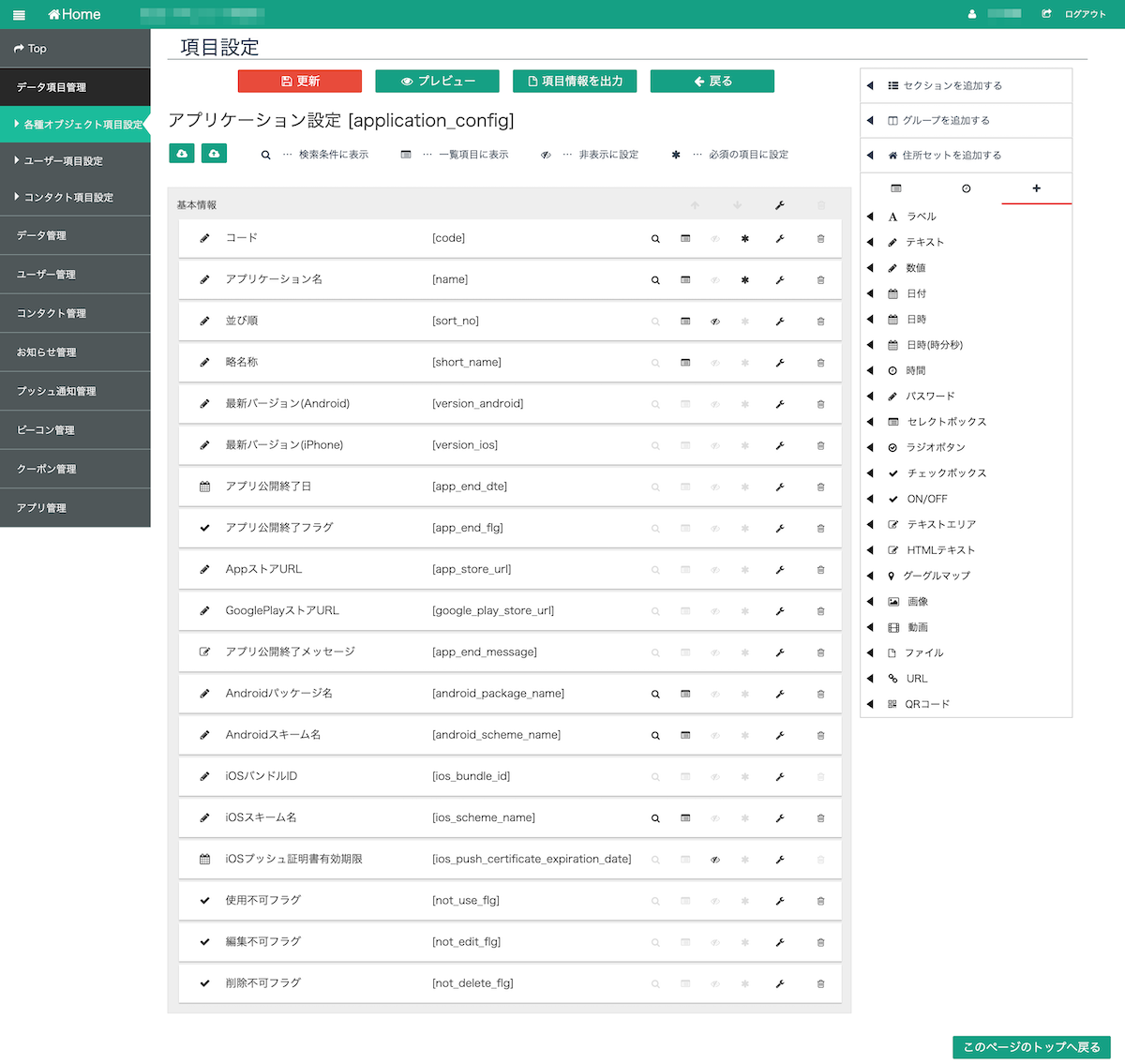
アプリケーション設定を取得する
アプリケーション設定の取得はgetApplicationSettingData
メソッドで⾏います。
RKZService.sharedInstance().getApplicationSettingData { appSettings, responseStatus in
if responseStatus.isSuccess, let appSettings = appSettings {
// 成功時
print("name:", appSettings.name ?? "") // アプリ名
print("version_android:", appSettings.version_android ?? "") // 最新バージョン(Android)
print("version_ios:", appSettings.version_ios ?? "") // 最新バージョン(iPhone)
print("app_store_url:", appSettings.app_store_url ?? "") // AppストアURL
print("google_play_store_url:", appSettings.google_play_store_url ?? "") // GooglePlayストアURL
print("app_end_dte:", appSettings.app_end_dte ?? "") // アプリ公開終了日
print("app_end_flg:", appSettings.app_end_flg) // アプリ公開終了フラグ
print("app_end_message:", appSettings.app_end_message ?? "") // アプリ公開終了メッセージ
print("site_url:", appSettings.attributes?["site_url"] ?? "") // 独自に追加した項目
} else {
// 失敗
print("statusCode:", responseStatus.statusCode.rawValue)
print("message:", responseStatus.message ?? "")
}
}
[[RKZService sharedInstance] getApplicationSettingDataWithBlock:^(RKZApplicationConfigData * _Nullable appSettings, RKZResponseStatus * _Nonnull responseStatus) {
if (responseStatus.isSuccess) {
// 成功時
NSLog(@"name: %@", appSettings.name); // アプリ名
NSLog(@"version_android: %@", appSettings.version_android); // 最新バージョン(Android)
NSLog(@"version_ios: %@", appSettings.version_ios); // 最新バージョン(iPhone)
NSLog(@"app_store_url: %@", appSettings.app_store_url); // AppストアURL
NSLog(@"google_play_store_url: %@", appSettings.google_play_store_url); // GooglePlayストアURL
NSLog(@"app_end_dte: %@", appSettings.app_end_dte); // アプリ公開終了日
NSLog(@"app_end_flg: %d", appSettings.app_end_flg); // アプリ公開終了フラグ
NSLog(@"app_end_message: %@", appSettings.app_end_message); // アプリ公開終了メッセージ
NSLog(@"site_url: %@", appSettings.attributes[@"site_url"]); // 独自に追加した項目
} else {
// 失敗
NSLog(@"statusCode: %ld", responseStatus.statusCode);
NSLog(@"message: %@", responseStatus.message);
}
}];
RKZClient.getInstance().getApplicationSettingData { appSettings, rkzResponseStatus ->
if (rkzResponseStatus.isSuccess) {
// 成功時
Log.d(TAG, "name: ${appSettings.name}") // アプリ名
Log.d(TAG, "versionAndroid: ${appSettings.versionAndroid}") // 最新バージョン(Android)
Log.d(TAG, "versionIos: ${appSettings.versionIos}") // 最新バージョン(iPhone)
Log.d(TAG, "appStoreUrl: ${appSettings.appStoreUrl}") // AppストアURL
Log.d(TAG, "googlePlayStoreUrl: ${appSettings.googlePlayStoreUrl}") // GooglePlayストアURL
Log.d(TAG, "appEndDte: ${appSettings.appEndDte}") // アプリ公開終了日
Log.d(TAG, "isAppEndFlg: ${appSettings.isAppEndFlg}") // アプリ公開終了フラグ
Log.d(TAG, "appEndMessage: ${appSettings.appEndMessage}") // アプリ公開終了メッセージ
Log.d(TAG, "site_url: ${appSettings.attributes["site_url"]}") // 独自に追加した項目
} else {
// 失敗
Log.e(TAG, "statusCode: ${rkzResponseStatus.statusCode}")
Log.e(TAG, "message: ${rkzResponseStatus.message}")
}
}
RKZClient.getInstance().getApplicationSettingData(new OnGetApplicationSettingDataListener() {
@Override
public void onGetApplicationSettingData(ApplicationConfig appSettings, RKZResponseStatus rkzResponseStatus) {
if (rkzResponseStatus.isSuccess()) {
// 成功時
Log.d(TAG, "name: " + appSettings.getName()); // アプリ名
Log.d(TAG, "versionAndroid: " + appSettings.getVersionAndroid()); // 最新バージョン(Android)
Log.d(TAG, "versionIos: " + appSettings.getVersionIos()); // 最新バージョン(iPhone)
Log.d(TAG, "appStoreUrl: " + appSettings.getAppStoreUrl()); // AppストアURL
Log.d(TAG, "googlePlayStoreUrl: " + appSettings.getGooglePlayStoreUrl()); // GooglePlayストアURL
Log.d(TAG, "appEndDte: " + appSettings.getAppEndDte()); // アプリ公開終了日
Log.d(TAG, "isAppEndFlg: " + appSettings.isAppEndFlg()); // アプリ公開終了フラグ
Log.d(TAG, "appEndMessage: " + appSettings.getAppEndMessage()); // アプリ公開終了メッセージ
Log.d(TAG, "site_url: " + appSettings.getAttributesValueString("site_url")); // 独自に追加した項目
} else {
// 失敗
Log.e(TAG, "statusCode: " + rkzResponseStatus.getStatusCode());
Log.e(TAG, "message: " + rkzResponseStatus.getMessage());
}
}
});
RKZClient.getApplicationSettingData(function (appSettings) {
// 成功時
console.debug('name:', appSettings.name) // アプリ名
console.debug('version_android:', appSettings.version_android) // 最新バージョン(Android)
console.debug('version_ios:', appSettings.version_ios) // 最新バージョン(iPhone)
console.debug('app_store_url:', appSettings.app_store_url) // AppストアURL
console.debug('google_play_store_url:', appSettings.google_play_store_url) // GooglePlayストアURL
console.debug('app_end_dte:', appSettings.app_end_dte) // アプリ公開終了日
console.debug('app_end_flg:', appSettings.app_end_flg) // アプリ公開終了フラグ
console.debug('app_end_message:', appSettings.app_end_message) // アプリ公開終了メッセージ
console.debug('site_url:', appSettings.attributes.site_url) // 独自に追加した項目
}, function (error) {
// 失敗時
console.error('status_code:', error.status_code)
console.error('message:', error.message)
})
const appSettings = await RKZ.appSettings()
console.debug('name:', appSettings.name) // アプリ名
console.debug('version_android:', appSettings.version_android) // 最新バージョン(Android)
console.debug('version_ios:', appSettings.version_ios) // 最新バージョン(iPhone)
console.debug('app_store_url:', appSettings.app_store_url) // AppストアURL
console.debug('google_play_store_url:', appSettings.google_play_store_url) // GooglePlayストアURL
console.debug('app_end_dte:', appSettings.app_end_dte) // アプリ公開終了日
console.debug('app_end_flg:', appSettings.app_end_flg) // アプリ公開終了フラグ
console.debug('app_end_message:', appSettings.app_end_message) // アプリ公開終了メッセージ
console.debug('site_url:', appSettings.attributes.site_url) // 独自に追加した項目
final appSettings = await RKZClient.instance.appSettings();
print('name: ${appSettings.name}'); // アプリ名
print('versionAndroid: ${appSettings.versionAndroid}'); // 最新バージョン(Android)
print('versionIos: ${appSettings.versionIos}'); // 最新バージョン(iPhone)
print('appStoreUrl: ${appSettings.appStoreUrl}'); // AppストアURL
print('googlePlayStoreUrl: ${appSettings.googlePlayStoreUrl}'); // GooglePlayストアURL
print('appEndDte: ${appSettings.appEndDte}'); // アプリ公開終了日
print('appEndFlg: ${appSettings.appEndFlg}'); // アプリ公開終了フラグ
print('appEndMessage: ${appSettings.appEndMessage}'); // アプリ公開終了メッセージ
print('site_url: ${appSettings.attributes?['site_url']}'); // 独自に追加した項目
ヒント
アプリケーションで独自に追加した項目はattributes
というプロパティに設定されて返ってきます。